Learn Multiplatform ARM Assembly
Programming... For the Future!
Multiplatform Lessons
In this series we look at code which does not rely on the hardware of a
specific system, these are useful code fragments which will work on any ARM
based system

|
Lesson M1 - Random Numbers and Ranges
Let's write some simple routines we may need in a game!
We'll write a psuedo random number generator, which can create
repeatable 16 bit random numbers.
We'll also create a 'Range checker' which can be used as a
collision detection routine for unsigned 8 bit numbers |
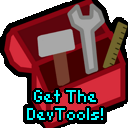 |
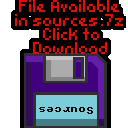
V1_Random.asm |
|
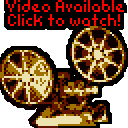 |
 |
The
Random number generator shown today is far from the 'Best'...
but it does work!
It's used in these tutorials by all the games as it's been
tested without any problems, and has been ported to many
different systems and CPUs, and given the same random seed, it
gives the same results... meaning it can be used for
generating random levels in your game, and the level will be
the same on every system.
|
Random number generation
To allow our random number
generator to create good varied results, we use a pair of 16
byte lookup tables |
 |
We have two random number generators,
The first returns a single byte in R0... using rotations and
EOR, using two 8 bit seeds R1/R4
|
|
We need the 'Low byte' to be very different, or the 16 bit
values won't be very random!
We use the lookup tables to get some new data, and combine these
to produce the second value |
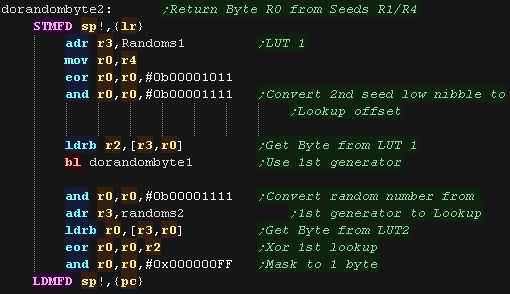 |
We have two routines we can use to get values
"DoRandomWord" will get a 16 bit value R3,R6 from seed R1, this
can be used to produce 'psuedorandom' data for times we want
repeatable results.
"DoRandom" is an easy function to return an 8 bit byte in R0...
it automatically updates its seed every time. |
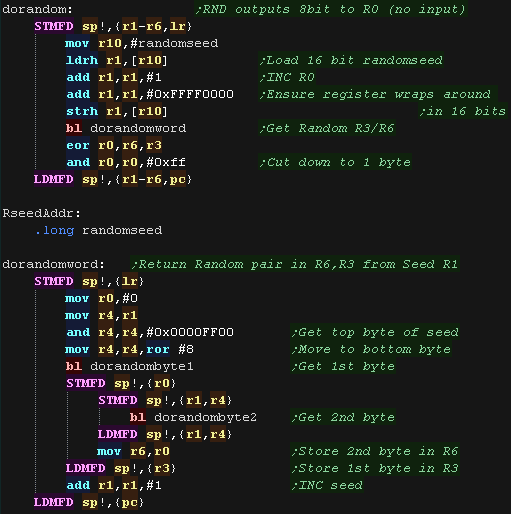 |
To
be usable, a random number generator needs to produce every
possible random number (0-255 or 0-65535)... otherwise you
could have serious problems with your program (if you're
waiting for a result that never happens).
The more 'random' the data the better... that is, if you
plotted the values on a graph there should be no patterns
present.
|
 |
Range checking
We need to do 'Collision detection'.
We specify a two targets and a 'range' ... if target
1 is within 'Range' of target
2, we return A=1... if it's out of range we return A=0...
This means we can do a BNE or BEQ on return. |
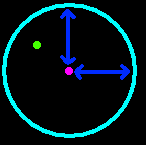 |
The range check is simple... we test X and Y axis
We test the X axis first.
we subtract the 'RANGE' from each
axis of the object, and check if we went
below Zero (if so that direction is over the limit)... we then
compare to the position... if it's lower
we're out of range... if not we need to test more!
Next we add 'RANGE' twice... once to
move back to the center and once to move to the right... we then
compare to the position...
If it's higher we're out of range... if not we need
to test the Y axis!
We repeat the same tests for the Y axis. |
|

|
Lesson M2 - BCD, Binary Coded Decimal!
In games we may wish to use binary coded decimal to store scores in
a way that's easy to convert to Ascii
Here we'll look at some simple routines to support BCD on ARM. |
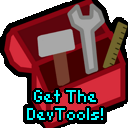 |
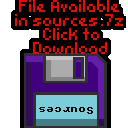
BCD_Test.asm
|
|
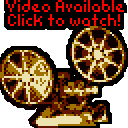 |
The Battle for BCD
Converting large numbers from Hex to Ascii is hard!
A common simple solution to this is Binary Coded Decimal... This
stores a single 0-9 digit in each nibble - Two per byte, This
is known as PACKED BCD!
This is easier to show to screen than normal hex numbers.
|
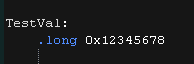
 |
BCD only uses a 0-9 value in each nibble... but of course each
nibble can really support 0-F
Suppose we repeatedly add 1 to the value 8... we'll need to correct
the value once the value goes over 9
values like $0A will need to be converted to the correct BCD value
of $10... and we'll need to do that ourselves! |
$08 +1
$09 +1
$0A -> $10 |
To ShowBCD, we need to step through each of
the 8 digits in our 32 bit value..... here we'll show R1 to the
screen
we rotate a digit (nibble) out of R1 into R0, add #48 to convert it
to Ascii, and show it to the screen with PrintChar
We repeat until all 8 characters are shown. |
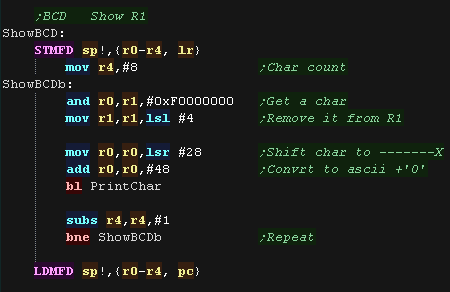 |
We'll need to AddBCD to add score... but this is
a little more tricky!
Here we're adding our values one digit at a time, but we need to
cope with any carry!...
If a digit goes over 9, we need to add 6, skipping over the A-F part
of the nibble, and storing any carry for the next digit in R6
we repeat for all 8 digits. |
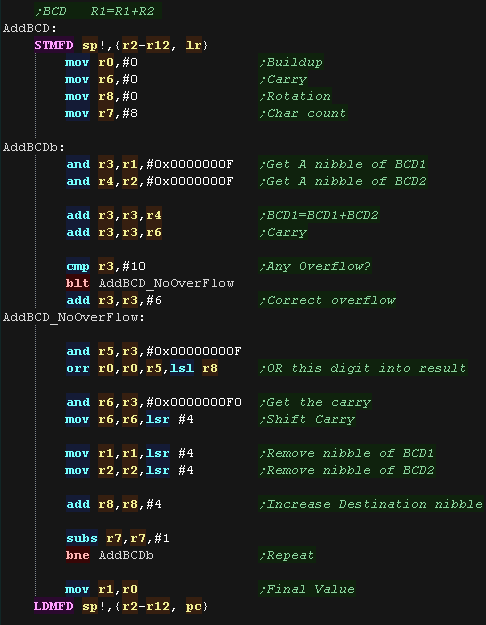 |
We can write SubBCD to subtract BCD in a
similar way
Once again we work through the digits keeping track of the carry. |
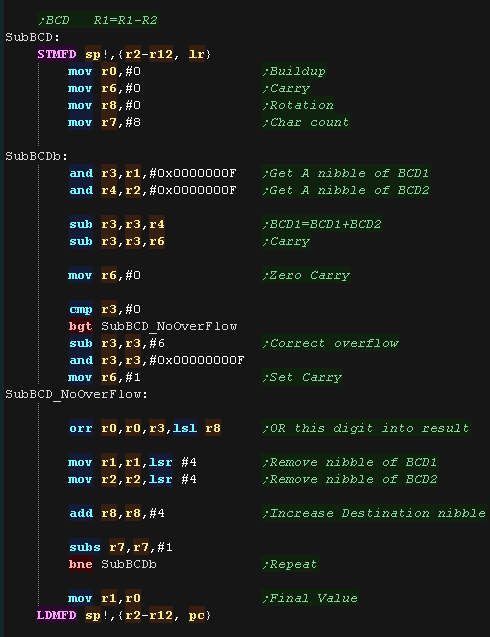 |
 |
We'll also need to compare two BCD values
for our highscore routine, but we don't need any special code to
do this, we can just use the normal CMP to do the job!
|
| |
Buy my Assembly programming book on Amazon in Print or Kindle!
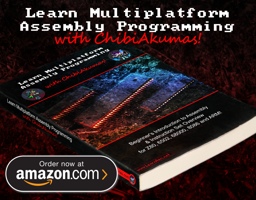
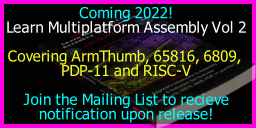
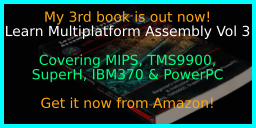
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
Buy my Assembly programming book on Amazon in Print or Kindle!
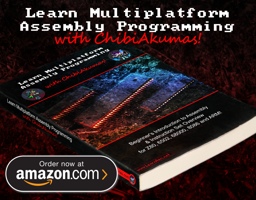
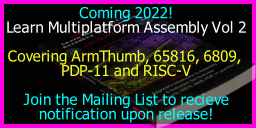
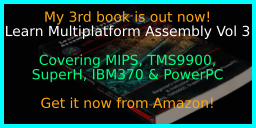
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
Buy my Assembly programming book on Amazon in Print or Kindle!
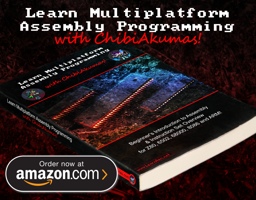
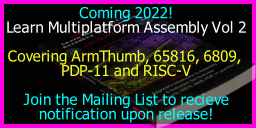
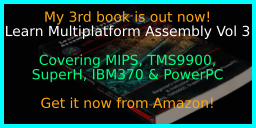
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
|